Summary: in this tutorial, you’ll learn about the PHP if elseif
statement to execute code blocks based on multiple boolean expressions.
Introduction to the PHP if elseif statement
The if
statement evaluates an expression
and executes a code block if the expression
is true:
<?php
if (expression) {
statement;
}
Code language: HTML, XML (xml)
The if
statement can have one or more optional elseif
clauses. The elseif
is a combination of if
and else
:
<?php
if (expression1) {
statement;
} elseif (expression2) {
statement;
} elseif (expression3) {
statement;
}
Code language: HTML, XML (xml)
PHP evaluates the expression1
and execute the code block in the if
clause if the expression1
is true
.
If the expression1
is false
, the PHP
evaluates the expression2
in the next elseif
clause. If the result is true
, then PHP executes the statement in that elseif
block. Otherwise, PHP evaluates the expression3
.
If the expression3
is true
, PHP executes the block that follows the elseif
clause. Otherwise, PHP ignores it.
Notice that when an if
statement has multiple elseif
clauses, the elseif
will execute only if the expression in the preceding if
or elseif
clause evaluates to false
.
The following flowchart illustrates how the if elseif
statement works:
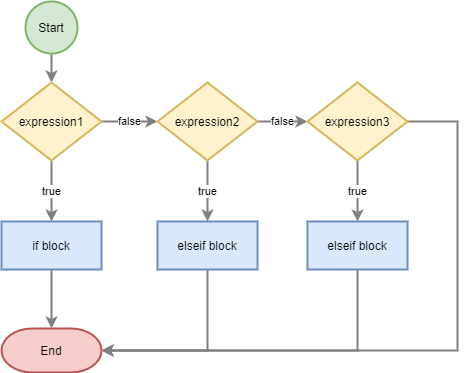
The following example uses the if elseif
statement to display whether the variable $x
is greater than $y
:
<?php
$x = 10;
$y = 20;
if ($x > $y) {
$message = 'x is greater than y';
} elseif ($x < $y) {
$message = 'x is less than y';
} else {
$message = 'x is equal to y';
}
echo $message;
Code language: HTML, XML (xml)
Output:
x is less than y
The script shows the message x is less than y
as expected.
PHP if elseif alternative syntax
PHP also supports an alternative syntax for the elseif
without using curly braces like the following:
<?php
if (expression):
statement;
elseif (expression2):
statement;
elseif (expression3):
statement;
endif;
Code language: HTML, XML (xml)
In this syntax:
- Use a semicolon (:) after each condition following the
if
orelseif
keyword. - Use the
endif
keyword instead of a curly brace (}
) at the end of theif
statement.
The following example uses the elseif
alternative syntax:
<?php
$x = 10;
$y = 20;
if ($x > $y) :
$message = 'x is greater than y';
elseif ($x < $y):
$message = 'x is less than y';
else:
$message = 'x is equal to y';
endif;
echo $message;
Code language: HTML, XML (xml)
The alternative syntax is suitable for use with HTML.
PHP elseif vs. else if
PHP allows you to write else if
(in two words) that has the same result as elseif
(in a single word):
<<?php
if (expression) {
statement;
} else if (expression2) {
statement2;
}
Code language: PHP (php)
The else if
in this case, is the same as the following nested if...else
structure:
if (expression) {
statement;
} else {
if (expression2) {
statement2;
}
}
Code language: JavaScript (javascript)
If you use the alternative syntax, you need to use the if...elseif
statement instead of the if...else if
statement. Otherwise, you’ll get an error.
The following example doesn’t work and cause an error:
<?php
$x = 10;
$y = 20;
if ($x > $y) :
echo 'x is greater than y';
else if ($x < $y):
echo 'x is equal to y';
else:
echo 'x is less than y';
endif;
Code language: HTML, XML (xml)
Summary
- Use the
if...elseif
statement to evaluate multiple expressions and execute code blocks conditionally. - Only the
if...elseif
supports alternative syntax, theif...else if
doesn’t. - Do use the
elseif
whenever possible to make your code more consistent.